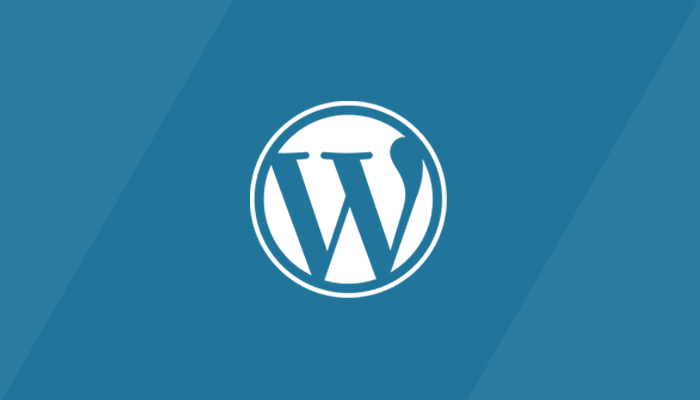
Useful Tricks for the WordPress Functions File
WordPress themes come with a powerful functions.php file. The functions.php file is where you add unique features to your WordPress theme. It can be used to hook into the core functions of WordPress to make your theme more modular, extensible, and functional.
This article is a collection of some of the most helpful tricks for your WordPress functions file.
1. Remove WordPress Version Number
You should always use the latest version of WordPress. However, you may still want to remove the WordPress version number from your site. Simply add this code snippet to your functions file.
function wpb_remove_version() { return ''; } add_filter('the_generator', 'wpb_remove_version');
2. Add a Custom Dashboard Logo
Want to white label your WordPress admin area? Adding a custom dashboard logo is the first step in the process.
First you’ll need to upload your custom logo to your theme’s images folder as custom-logo.png. Make sure your custom logo is 16×16 pixels in size.
After that you can add this code to your theme’s functions file.
function wpb_custom_logo() { echo ' <style type="text/css"> #wpadminbar #wp-admin-bar-wp-logo > .ab-item .ab-icon:before { background-image: url(' . get_bloginfo('stylesheet_directory') . '/images/custom-logo.png) !important; background-position: 0 0; color:rgba(0, 0, 0, 0); } #wpadminbar #wp-admin-bar-wp-logo.hover > .ab-item .ab-icon { background-position: 0 0; } </style> '; } //hook into the administrative header output add_action('wp_before_admin_bar_render', 'wpb_custom_logo');
3. Hide admin bar (during development)
show_admin_bar( false );
4. Hide login errors
Hide the login errors/hints and replace them with a simple message.
function hide_login_errors(){ return 'Oepsss, you broke me!'; } add_filter( 'login_errors' , 'hide_login_errors' );
5. Disable XML-RPC
If you dont want to access and publish to your blog remotely you should disable this. This will also completely disable the whole XML-RPC class.
add_filter( 'wp_xmlrpc_server_class' , '__return_false' ); add_filter( 'xmlrpc_enabled' , '__return_false' );
6. Auto update wordpress
This will auto update your WordPress core (big and minor updates).
define( 'WP_AUTO_UPDATE_CORE' , true );
7. Auto update all plugins
Auto update all of your plugins.
define( 'WP_AUTO_UPDATE_CORE' , true );
8. Auto update all themes
Auto update all of your themes.
add_filter( 'auto_update_theme' , '__return_true' );
9. Change the Default Gravatar in WordPress
Have you seen the default mystery man avatar on blogs? You can easily replace it with your own branded custom avatars. Simply upload the image you want to use as default avatar and then add this code to your functions file.
function wpb_new_gravatar ($avatar_defaults) { $myavatar = 'http://example.com/wp-content/uploads/2017/01/wpb-default-gravatar.png'; $avatar_defaults[$myavatar] = "Default Gravatar"; return $avatar_defaults; } add_filter( 'avatar_defaults', 'wpb_new_gravatar' );
10. Colors convert to rgba
Give function hex code (e.g. #eeeeee), returns array of RGB values.
function hexrgb($color){ if (isset($color[0])&&$color[0] == '#') $color = substr($color, 1); if (strlen($color) == 6) list($r, $g, $b) = array($color[0].$color[1], $color[2].$color[3], $color[4].$color[5]); elseif (strlen($color) == 3) list($r, $g, $b) = array($color[0].$color[0], $color[1].$color[1], $color[2].$color[2]); else return false; $r = hexdec($r); $g = hexdec($g); $b = hexdec($b); return $r.', '.$g.', '.$b; }
11. Remove Default Image Links in WordPress
By default, when you uplaod an image in WordPress it is automatically linked to the image file or the attachment page. If users click on the image they are then taken to a new page away from your post.
Here is how you can easily stop WordPress from automatically linking image uploads. All you have to do is to add this code snippet to your functions file:
function wpb_imagelink_setup() { $image_set = get_option( 'image_default_link_type' ); if ($image_set !== 'none') { update_option('image_default_link_type', 'none'); } } add_action('admin_init', 'wpb_imagelink_setup', 10);
12. Replace default WordPress jQuery script with Google Library
If you are sure that you want to change the core jQuery
version, in that case you may add the following code in your active theme’s functions.php
file.
//Making jQuery Google API function modify_jquery() { if (!is_admin()) { wp_deregister_script('jquery-core'); wp_register_script('jquery-core', 'http://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js', true, '1.11.3'); wp_enqueue_script('jquery-core'); wp_deregister_script('jquery-migrate'); wp_register_script('jquery-migrate', 'http://cdn.yourdomain.com/wp-includes/js/jquery/jquery-migrate.min.js', true, '1.2.1'); wp_enqueue_script('jquery-migrate'); } } add_action( 'init', 'register_jquery' );
13. Change category checkbox to radio in Cases post formate
add_action('add_meta_boxes','bptproject_add_meta_boxes',10,2); function bptproject_add_meta_boxes($post_type, $post) { if ('bptproject'==$post_type) { ob_start(); } } add_action('dbx_post_sidebar','bptproject_dbx_post_sidebar'); function bptproject_dbx_post_sidebar() { $html = ob_get_clean(); $html = str_replace('"checkbox"','"radio"',$html); echo $html; }
14. Disable support for comments and trackbacks in post types
function df_disable_comments_post_types_support() { $post_types = get_post_types(); foreach ($post_types as $post_type) { if(post_type_supports($post_type, 'comments')) { remove_post_type_support($post_type, 'comments'); remove_post_type_support($post_type, 'trackbacks'); } } } add_action('admin_init', 'df_disable_comments_post_types_support');
15. Close comments on the front-end
function df_disable_comments_status() { return false; } add_filter('comments_open', 'df_disable_comments_status', 20, 2); add_filter('pings_open', 'df_disable_comments_status', 20, 2);
16. Hide existing comments
function df_disable_comments_hide_existing_comments($comments) { $comments = array(); return $comments; } add_filter('comments_array', 'df_disable_comments_hide_existing_comments', 10, 2);
17. Remove comments page in menu
function df_disable_comments_admin_menu() { remove_menu_page('edit-comments.php'); } add_action('admin_menu', 'df_disable_comments_admin_menu');
18. Redirect any user trying to access comments page
function df_disable_comments_admin_menu_redirect() { global $pagenow; if ($pagenow === 'edit-comments.php') { wp_redirect(admin_url()); exit; } } add_action('admin_init', 'df_disable_comments_admin_menu_redirect');
19.Remove comments metabox from dashboard
function df_disable_comments_dashboard() { remove_meta_box('dashboard_recent_comments', 'dashboard', 'normal'); } add_action('admin_init', 'df_disable_comments_dashboard');
20. Remove comments links from admin bar
function df_disable_comments_admin_bar() { if (is_admin_bar_showing()) { remove_action('admin_bar_menu', 'wp_admin_bar_comments_menu', 60); } } add_action('init', 'df_disable_comments_admin_bar');
21. hide the comment icon in the admin bar
add_action( 'admin_bar_menu', 'clean_admin_bar', 999 ); function clean_admin_bar( $wp_admin_bar ) { $wp_admin_bar->remove_node( 'comments' ); }
Synthi at 4:49 pm
thanks you for these useful tricks
Andrew at 6:31 pm
Great post!!!
I am a newbie and looking for such tricks.
Thanks.
OE at 3:23 am
How to use these above with child theme functions file?
BuyProTheme at 5:12 am
Nothing difference with parent or child theme’s functions.php
kadirzy at 3:23 am
Excellent post – thank you for the tips & tricks. They are all very helpful
Reitz at 4:42 am
Thanks for this codes.